Hardware components | ||||||
![]() |
| × | 1 | |||
| × | 1 | ||||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
| × | 1 | ||||
| × | 1 | ||||
| × | 1 | ||||
Software apps and online services | ||||||
![]() |
| |||||
|
This project proposes a photovoltaic (PV) model for the design of PV systems with a simple MPPT to achieve high efficiency, faster response and low cost. First, a PV panel model is developed using SPICE code in Proteus tool. The verification and the validation are performed via an experimental test bench based on Arduino board. Afterwards, Both methods (Incremental conductance and Perturb & observe) are implemented in the low-cost Arduino Uno board using the simulated PV panel model. To validate our system, a hardware testbench is implemented using the low-cost ATMega328 microcontroller in the Arduino Uno board. Substantial cost reduction has been attained proving the financial competitiveness of the proposed controller.
This project is linked to this research paper.
Download data from here.
Please, refer to this presentation to get an overview about this system. Note: Please, use google chrome toread this presentation.
Proteus PV panel model simulation
• Please, go to graph menu and click on simulate graph as follows:
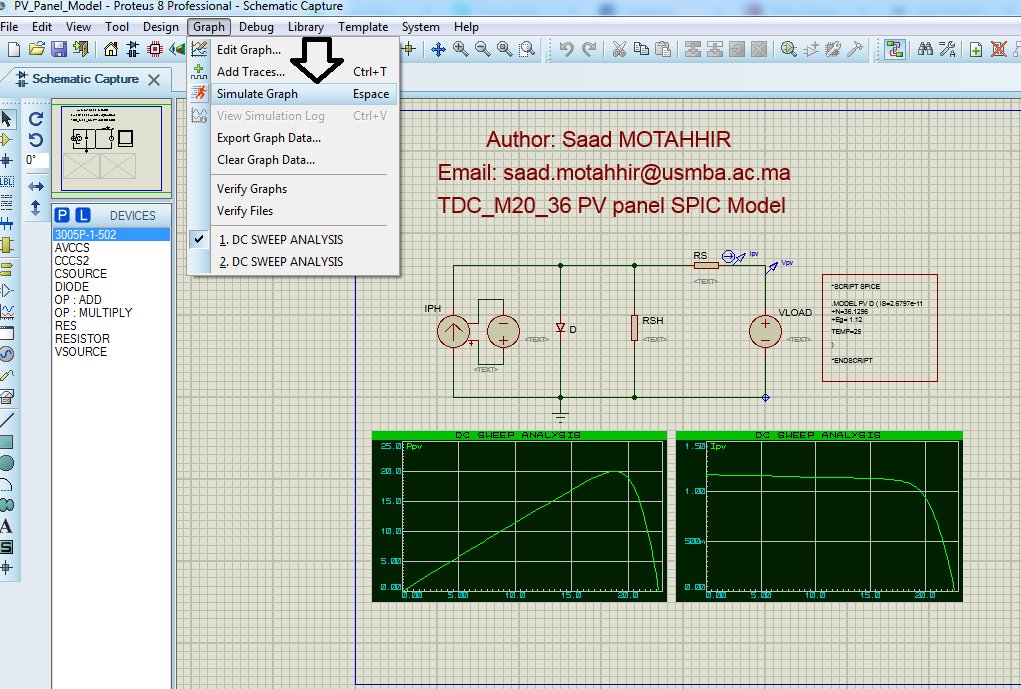
Add Arduino into Proteus:
• These two files are named as ArduinoTEP.LIB and ArduinoTEP.IDX.
• Copy these two files and place them in the libraries folder of your Proteus software.
• Now, restart your Proteus software and in components section search for ArduinoTEP as shown in below figure:
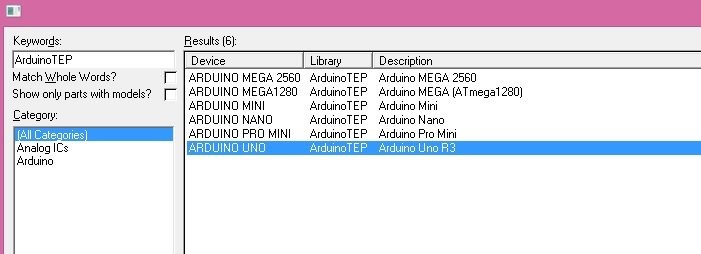
Simulate the MPPT controller in Proteus under stable irradiance
• Load the .hex file in the Arduino Uno.
• Click on play to simulate the PV system or go to graph menu and click on simulate graph to generate Ppv(t) curve.
Simulate the MPPT controller in Proteus under variable irradiance
• Load the .hex file in the Arduino Uno.
• Click on play to simulate the PV system or go to graph menu and click on simulate graph to generate Ppv(t) curve.
Costless and effective Embedded system based control for PV system
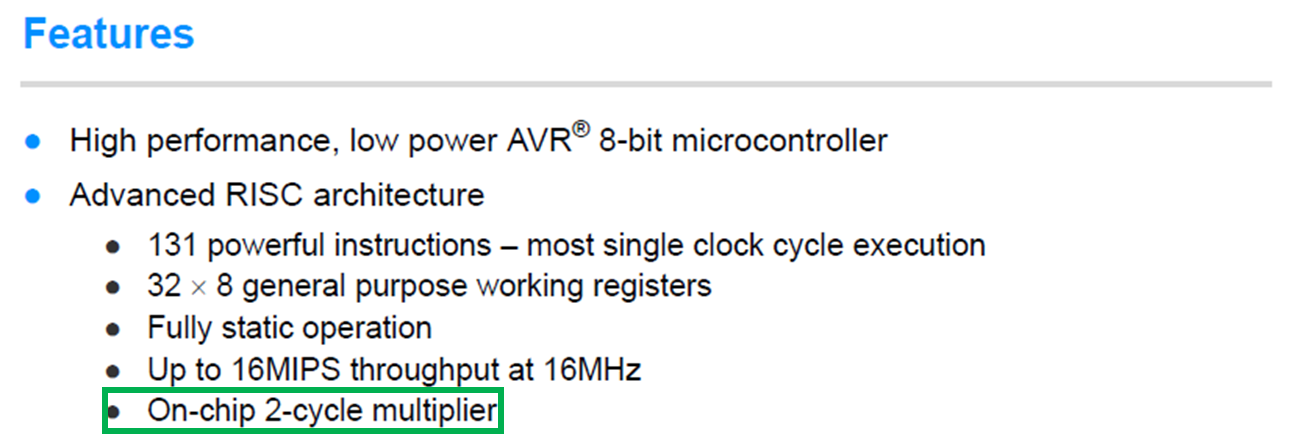
Experimental results
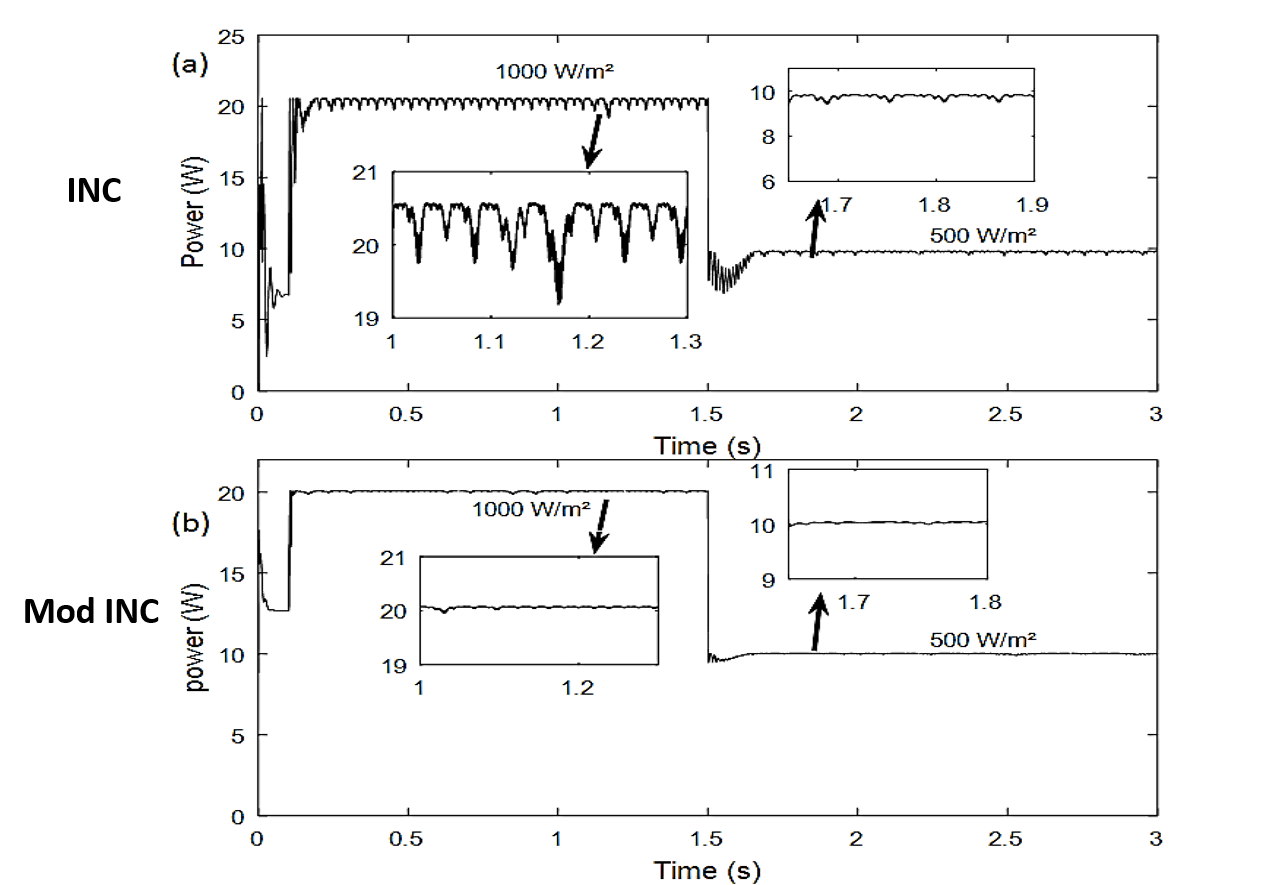
PandO_Code.ino
C/C++/*****************************************************************************
Copyright Motahhir All Rights Reserved
*****************************************************************************/
/*****************************************************************************
Header Files included
*****************************************************************************/
/******************************************************************************
PROJECT : MPPT (P&O) implementation
Function : P&O Arduino Code
******************************************************************************
* *
Written by : Saad Motahhir Date : 09/10/2016
* *
Email : saad.motahhir@usmba.ac.ma
******************************************************************************
MODIFICATION LOG:
******************************************************************************
Modified by : Date :
Comments :
******************************************************************************/
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
float sensorValue1 = 0;
float sensorValue2 = 0;
float voltageValue = 0;
float currentValue = 0;
float Power_now = 0, Power_anc = 0, voltage_anc = 0;
float delta = 3;
float pwm = 128;
void setup()
{
pinMode(6, OUTPUT);
lcd.begin(16, 2);
}
void loop()
{
sensorValue1 = analogRead(A0);
sensorValue2 = analogRead(A1);
voltageValue = (sensorValue1 * 5.0 / 1023.0) * 5;
currentValue = (sensorValue2 * 5.0 / 1023.0);
lcd.setCursor(0, 0);
Power_now = voltageValue * currentValue;
lcd.print("Ppv=");
lcd.print(Power_now);
lcd.print("W");
lcd.print(pwm);
lcd.setCursor(0, 1);
lcd.print("V=");
lcd.print(voltageValue);
lcd.print("V I=");
lcd.print(currentValue);
lcd.print("A");
if (Power_now > Power_anc)
{ if (voltageValue > voltage_anc)
pwm = pwm - delta;
else
pwm = pwm + delta;
}
else
{
if (voltageValue > voltage_anc)
pwm = pwm + delta;
else
pwm = pwm - delta;
}
Power_anc = Power_now;
voltage_anc = voltageValue;
if (pwm < 20)
pwm = 20;
if (pwm > 150)
pwm = 150;
analogWrite(6, pwm);
}
INC_Code.ino
C/C++/*****************************************************************************
Copyright Motahhir All Rights Reserved
*****************************************************************************/
/*****************************************************************************
Header Files included
*****************************************************************************/
/******************************************************************************
PROJECT : MPPT (INC) implementation
Function : INC Arduino Code
******************************************************************************
* *
Written by : Saad Motahhir Date : 09/10/2016
* *
Email : saad.motahhir@usmba.ac.ma
******************************************************************************
MODIFICATION LOG:
******************************************************************************
Modified by : Date :
Comments :
******************************************************************************/
#include <LiquidCrystal.h>
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
float sensorValue1 = 0;
float sensorValue2 = 0;
float voltageValue = 0;
float currentValue = 0;
float Power_now = 0, Power_anc=0, Current_anc =0,Voltage_anc=0, deltaI=0, deltaV=0 ;
float delta = 1.4;
float pwm = 128;
void setup()
{
pinMode(6, OUTPUT);
lcd.begin(16, 2);
}
void loop()
{
sensorValue1 = analogRead(A0);
sensorValue2 = analogRead(A1);
voltageValue= (sensorValue1 * 5.0 /1023.0) *5;
currentValue= (sensorValue2 * 5.0 /1023.0);
lcd.setCursor(0, 0);
Power_now = voltageValue * currentValue;
lcd.print("Ppv=");
lcd.print(Power_now);
lcd.print("W");
lcd.setCursor(0, 1);
lcd.print("V=");
lcd.print(voltageValue);
lcd.print("V I=");
lcd.print(currentValue);
lcd.print("A");
deltaI= currentValue-Current_anc;
deltaV= voltageValue-Voltage_anc;
if(deltaV==0)
{ if(deltaI==0)
{// nothing to do
}
else
{ if(deltaI>0)
pwm=pwm-delta;
else
pwm=pwm+delta;
}
}
else
{ if((voltageValue*deltaI)+(currentValue*deltaV)==0)
{// nothing to do
}
else
{ if((deltaI/deltaV)+(currentValue/voltageValue)>0)
pwm=pwm-delta;
else
pwm=pwm+delta;
}
}
Voltage_anc= voltageValue;
Current_anc= currentValue;
Power_anc=Power_now;
if(pwm > 240)
pwm=240;
if (pwm < 15)
pwm=15;
analogWrite(6, pwm);
}
Comments