Hardware components | ||||||
| × | 1 | ||||
![]() |
| × | 6 | |||
![]() |
| × | 3 | |||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
Software apps and online services | ||||||
![]() |
| |||||
![]() |
| |||||
| ||||||
|
Temperature Sensor (Bithal): The sensor was used to to monitor and control temperature levels of the unfiltered water in the sewage treatment which then can be used to reduce bacteria's capacity to flocculate sediment, damage filter filaments, and inhibit the growth of specific bacteria.
Water level sensor (Abdullah) :The water level sensor was used to send alerts that indicates if the water level is high, medium, or low, in response to that the servo motor receives this signal as an input to do a programmed action either by opening or closing the valve.
Micro servo motor (Aref): The micro servo motor was used to open and close the gate for the water that goes to fill the tank. It opens from 0 to 180 degrees, and it receives the signals from the water level sensor, so once the tank is low, it will show a red LED, and the servo will open the gate to fill the water. If the LED is green, that means the tank is full, and the servo will close the gate to prevent water flow.
PH Sensor (Joy) : using the water sensor we measure the PH level of the water to insure it is within safe operating levels for irrigation and to insure the good bacteria in the solution do not die from the acidity of the base of the water.
Display Interface(Ivy) : as shown in the project, the system involves a lot of LEDs. To properly understand what is going on, a Bluetooth module was incorporated. This allows anyone with a Bluetooth-enabled device to connect to the system and see what is happening with water levels, PH, and temperature in real time.
Water level Sensor ( simulated circuit )
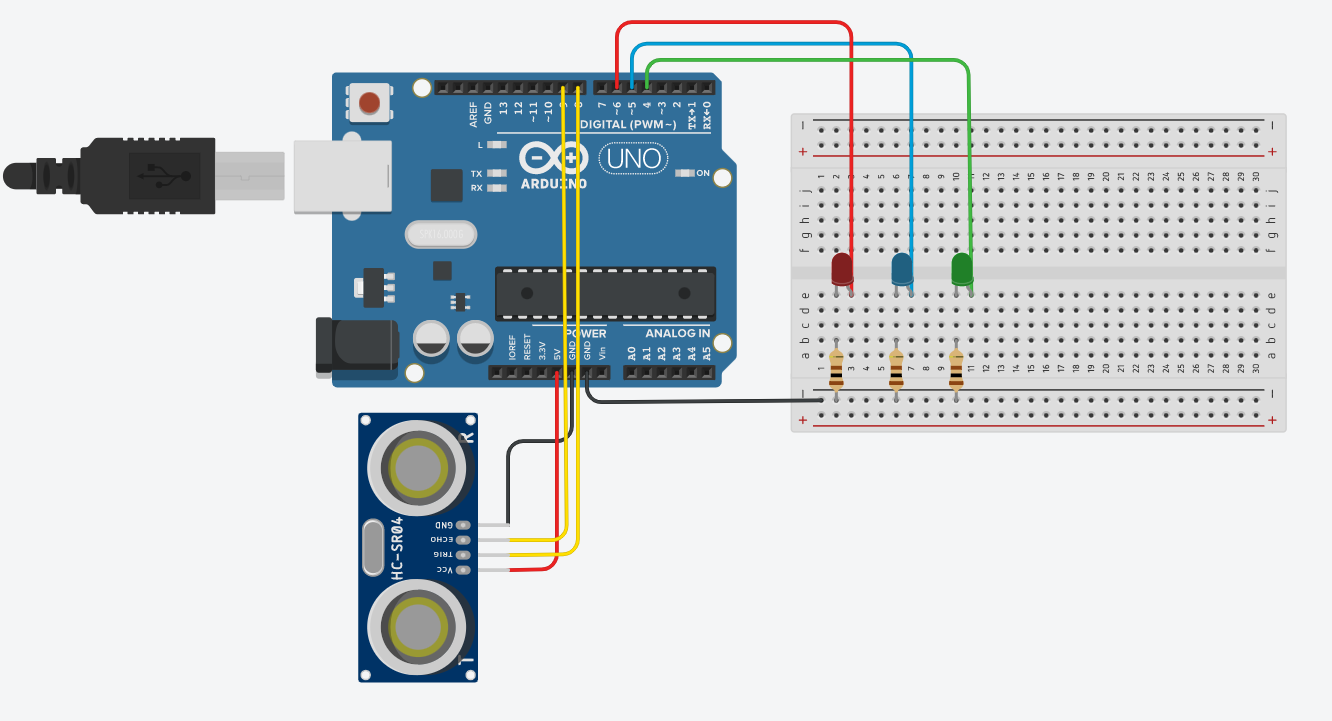
#include <OneWire.h>
#include <DallasTemperature.h>
#define ONE_WIRE_BUS 2
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
float Celsius = 0;
float Fahrenheit = 0;
void setup() {
//BITHAL
sensors.begin();
Serial.begin(9600);
pinMode(3, OUTPUT);
pinMode(4, OUTPUT);
pinMode(5, OUTPUT);
Serial.begin(9600);
pinMode(sensorPower, OUTPUT);
digitalWrite(sensorPower, LOW);
// Set LED pins as an OUTPUT
pinMode(redLED, OUTPUT);
pinMode(yellowLED, OUTPUT);
pinMode(greenLED, OUTPUT);
}
void loop() {
sensors.requestTemperatures();
Celsius = sensors.getTempCByIndex(0);
Fahrenheit = sensors.toFahrenheit(Celsius);
Serial.print(Celsius);
Serial.print(" C ");
Serial.print(Fahrenheit);
Serial.println(" F");
if (Celsius <= 35 && Celsius >= 20) {
digitalWrite(5, HIGH);
digitalWrite(3, LOW);
digitalWrite(4, LOW);
}
if (Celsius < 37 && Celsius > 35) {
digitalWrite(3, LOW);
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
}
if (Celsius < 20 && Celsius > 17) {
digitalWrite(3, LOW);
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
}
if (Celsius <= 100 && Celsius > 37) {
digitalWrite(4, LOW);
digitalWrite(5, LOW);
digitalWrite(3, HIGH);
}
if (Celsius < 17 && Celsius >= 0) {
digitalWrite(4, LOW);
digitalWrite(5, LOW);
digitalWrite(3, HIGH);
}
delay(1000);
}
/* Water level Sensor code
/* Calibration values */
int lowerThreshold = 420;
int upperThreshold = 520;
// Sensor pins
#define sensorPower 9
#define sensorPin A0
// Value for storing water level
int val = 0;
// Declare pins to which LEDs are connected
int redLED = 6;
int yellowLED = 7;
int greenLED = 8;
void setup() {
Serial.begin(9600);
pinMode(sensorPower, OUTPUT);
digitalWrite(sensorPower, LOW);
// Set LED pins as an OUTPUT
pinMode(redLED, OUTPUT);
pinMode(yellowLED, OUTPUT);
pinMode(greenLED, OUTPUT);
// Initially turn off all LEDs
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, LOW);
}
void loop() {
int level = readSensor();
if (level == 0) {
Serial.println("Water Level: Empty");
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, LOW);
}
else if (level > 0 && level <= lowerThreshold) {
Serial.println("Water Level: Low");
digitalWrite(redLED, HIGH);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, LOW);
}
}
else if (level > lowerThreshold && level <= upperThreshold) {
Serial.println("Water Level: Medium");
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, HIGH);
digitalWrite(greenLED, LOW);
}
else if (level > upperThreshold) {
Serial.println("Water Level: High");
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, HIGH);
}
delay(1000);
}
//This is a function used to get the reading
int readSensor() {
digitalWrite(sensorPower, HIGH);
delay(10);
val = analogRead(sensorPin);
digitalWrite(sensorPower, LOW);
return val;
}
#include <Servo.h>
int servoPin = 11;
Servo servo;
int angle = 0; // servo position in degrees
void setup() {
servo.attach(servoPin);
}
void loop() {
// scan from 0 to 180 degrees
for(angle = 0; angle < 180; angle++) {
servo.write(angle);
delay(15);
}
// now scan back from 180 to 0 degrees
for(angle = 180; angle > 0; angle--) {
servo.write(angle);
delay(15);
}
}
#define SensorPin A2 //pH meter Analog output to Arduino Analog Input 0
#define Offset 15.76 //deviation compensate
#define LED 13
#define samplingInterval 20
#define printInterval 800
#define ArrayLenth 40 //times of collection
#define uart Serial
int pHArray[ArrayLenth]; //Store the average value of the sensor feedback
int pHArrayIndex = 0;
void setup(void)
{
pinMode(LED, OUTPUT);
uart.begin(9600);
uart.println("pH meter experiment!"); //Test the uart monitor
}
void loop(void)
{
static unsigned long samplingTime = millis();
static unsigned long printTime = millis();
static float pHValue, voltage;
if (millis() - samplingTime > samplingInterval)
{
pHArray[pHArrayIndex++] = analogRead(SensorPin);
if (pHArrayIndex == ArrayLenth)pHArrayIndex = 0;
voltage = avergearray(pHArray, ArrayLenth) * 5.0 / 1024;
pHValue = -5.882 * voltage + Offset;
samplingTime = millis();
}
if (millis() - printTime > printInterval) //Every 800 milliseconds, print a numerical, convert the state of the LED indicator
{
uart.print("Voltage:");
uart.print(voltage, 2);
uart.print(" pH value: ");
uart.println(pHValue, 2);
digitalWrite(LED, digitalRead(LED) ^ 1);
printTime = millis();
}
}
double avergearray(int* arr, int number) {
int i;
int max, min;
double avg;
long amount = 0;
if (number <= 0) {
uart.println("Error number for the array to avraging!/n");
return 0;
}
if (number < 5) { //less than 5, calculated directly statistics
for (i = 0; i < number; i++) {
amount += arr[i];
}
avg = amount / number;
return avg;
} else {
if (arr[0] < arr[1]) {
min = arr[0]; max = arr[1];
}
else {
min = arr[1]; max = arr[0];
}
for (i = 2; i < number; i++) {
if (arr[i] < min) {
amount += min; //arr<min
min = arr[i];
} else {
if (arr[i] > max) {
amount += max; //arr>max
max = arr[i];
} else {
amount += arr[i]; //min<=arr<=max
}
}//if
}//for
avg = (double)amount / (number - 2);
}//if
return avg;
}
Bluetooth module
Arduino#include <SoftwareSerial.h>
SoftwareSerial mySerial(10, 11); // RX, TX
char w;
void setup() {
Serial.begin(9600);
mySerial.begin(9600);
delay(1000); //wait to start the device properly
}
void loop() {
if (mySerial.available()) {
w = mySerial.read();
Serial.println(w); //PC
delay(10);
//commands with Serial.println(); show on pc serial monitor
}
if (Serial.available()) {
w = Serial.read();
mySerial.println(w); //Phone
delay(10);
//commands with mySerial.println(); show on the device app
}
//shown on pc
Serial.println("This is a test run");
Serial.println("Congratulations! Device Connected!");
Serial.println();
//shown on device app
mySerial.println("This is a test run");
mySerial.println("Congratulations! Device Connected!");
mySerial.println();
delay(2000);
}
#include <OneWire.h>
#include <DallasTemperature.h>
#include <Servo.h>
#include <SoftwareSerial.h>
#define ONE_WIRE_BUS 2
#define sensorPower 9
#define sensorPin A0
#define SensorPin A2 //pH meter Analog output to Arduino Analog Input 0
#define Offset 15.76 //deviation compensate
#define LED 13
#define samplingInterval 20
#define printInterval 800
#define ArrayLenth 40 //times of collection
#define uart Serial
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
SoftwareSerial mySerial(10, 11); // RX, TX
char w;
float Celsius = 0;
float Fahrenheit = 0;
/* Water level Sensor code
/* Change these values based on your calibration values */
int lowerThreshold = 420;
int upperThreshold = 520;
// Value for storing water level
int val = 0;
int ran=0;
int ok =0;
int pHArray[ArrayLenth]; //Store the average value of the sensor feedback
int pHArrayIndex = 0;
Servo servo;
int angle = 0; // servo position in degrees
// Declare pins to which LEDs are connected
int redLED = 6;
int yellowLED = 7;
int greenLED = 8;
//Aref
int servoPin = 11;
void setup() {
pinMode(LED, OUTPUT);
uart.begin(9600);
uart.println("pH meter experiment!");
//Aref
servo.attach(servoPin);
//Abdullah
Serial.begin(9600);
pinMode(sensorPower, OUTPUT);
digitalWrite(sensorPower, LOW);
// Set LED pins as an OUTPUT
pinMode(redLED, OUTPUT);
pinMode(yellowLED, OUTPUT);
pinMode(greenLED, OUTPUT);
// Initially turn off all LEDs
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, LOW);
sensors.begin();
Serial.begin(9600);
pinMode(3, OUTPUT);
pinMode(4, OUTPUT);
pinMode(5, OUTPUT);
Serial.begin(9600);
mySerial.begin(9600);
delay(1000); //wait to start the device properly
}
void loop() {
int level = readSensor();
sensors.requestTemperatures();
Celsius = sensors.getTempCByIndex(0);
Fahrenheit = sensors.toFahrenheit(Celsius);
Serial.print(Celsius);
Serial.print(" C ");
Serial.print(Fahrenheit);
Serial.println(" F");
static unsigned long samplingTime = millis();
static unsigned long printTime = millis();
static float pHValue, voltage;
if (level == 0) {
Serial.println("Water Level: Empty");
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, LOW);
}
else if (level > 0 && level <= lowerThreshold) {
Serial.println("Water Level: Low");
digitalWrite(redLED, HIGH);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, LOW);
if (ran ==0){
for(angle = 0; angle < 180; angle++) {
servo.write(angle);
delay(15);
}
ran+=1;
ok=0;
}
}
else if (level > lowerThreshold && level <= upperThreshold) {
Serial.println("Water Level: Medium");
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, HIGH);
digitalWrite(greenLED, LOW);
}
else if (level > upperThreshold) {
Serial.println("Water Level: High");
digitalWrite(redLED, LOW);
digitalWrite(yellowLED, LOW);
digitalWrite(greenLED, HIGH);
if(ok==0){
for(angle = 180; angle > 0; angle--) {
servo.write(angle);
delay(15);
}
ok+=1;
ran =0;
}
}
if (Celsius <= 35 && Celsius >= 20) {
digitalWrite(5, HIGH);
digitalWrite(3, LOW);
digitalWrite(4, LOW);
}
if (Celsius <= 37 && Celsius > 35) {
digitalWrite(3, LOW);
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
}
if (Celsius < 20 && Celsius >= 17) {
digitalWrite(3, LOW);
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
}
if (Celsius <= 100 && Celsius > 37) {
digitalWrite(4, LOW);
digitalWrite(5, LOW);
digitalWrite(3, HIGH);
}
if (Celsius < 17 && Celsius >= 0) {
digitalWrite(4, LOW);
digitalWrite(5, LOW);
digitalWrite(3, HIGH);
}
delay(1000);
if (millis() - samplingTime > samplingInterval)
{
pHArray[pHArrayIndex++] = analogRead(SensorPin);
if (pHArrayIndex == ArrayLenth)pHArrayIndex = 0;
voltage = avergearray(pHArray, ArrayLenth) * 4.48 / 1024;
pHValue = -5.882 * voltage + Offset;
samplingTime = millis();
}
if (millis() - printTime > printInterval) //Every 800 milliseconds, print a numerical, convert the state of the LED indicator
{
uart.print("Voltage:");
uart.print(voltage, 2);
uart.print(" pH value: ");
uart.println(pHValue, 2);
digitalWrite(LED, digitalRead(LED) ^ 1);
printTime = millis();
}
if (mySerial.available()) {
w = mySerial.read();
Serial.println(w); //PC
delay(10);
//commands with Serial.println(); show on pc serial monitor
}
if (Serial.available()) {
w = Serial.read();
mySerial.println(w); //Phone
delay(10);
//commands with mySerial.println(); show on the device app
}
//shown on pc
Serial.println("This is a test run");
Serial.println("Congratulations! Device Connected!");
Serial.println();
//shown on device app
mySerial.println("This is a test run");
mySerial.println("Congratulations! Device Connected!");
mySerial.println();
delay(2000);
}
//This is a function used to get the reading
int readSensor() {
digitalWrite(sensorPower, HIGH);
delay(10);
val = analogRead(sensorPin);
digitalWrite(sensorPower, LOW);
return val;
}
double avergearray(int* arr, int number) {
int i;
int max, min;
double avg;
long amount = 0;
if (number <= 0) {
uart.println("Error number for the array to avraging!/n");
return 0;
}
if (number < 5) { //less than 5, calculated directly statistics
for (i = 0; i < number; i++) {
amount += arr[i];
}
avg = amount / number;
return avg;
} else {
if (arr[0] < arr[1]) {
min = arr[0]; max = arr[1];
}
else {
min = arr[1]; max = arr[0];
}
for (i = 2; i < number; i++) {
if (arr[i] < min) {
amount += min; //arr<min
min = arr[i];
} else {
if (arr[i] > max) {
amount += max; //arr>max
max = arr[i];
} else {
amount += arr[i]; //min<=arr<=max
}
}//if
}//for
avg = (double)amount / (number - 2);
}//if
return avg;
}
Comments