Hardware components | ||||||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
| × | 1 | ||||
| × | 1 | ||||
| × | 1 | ||||
Software apps and online services | ||||||
![]() |
| |||||
![]() |
| |||||
Hand tools and fabrication machines | ||||||
![]() |
|
Intelligent actuator that uses Azure Stream Analytics and Machine Learning to report back its own health status, preventing loss of time and money spent diagnosing problems. This allows you to replace your hardware before it crashes or dies.
Most importantly, in this project we were able to listen to the control commands from EventHub and utilize the power of Stream Analytics in remote monitoring and predictive maintenance. In short, we achieved two-way data transfer, to and from Raspberry Pi and Azure Cloud. This project is a basic demonstration of how this data transfer can be achieved. Trust us, it wasn't easy.
Some of the parts used in this project...
Raspberry Pi 3B with Andoer NEO-6M GPS module attached via serial.
Raspberry Pi 2B with Fez-Hat on top.
Hi-Tec HS-645MG Servos.
Raspberry Pi 2B with Fez-Hat on top.
Hi-Tec HS-645MG Servos.
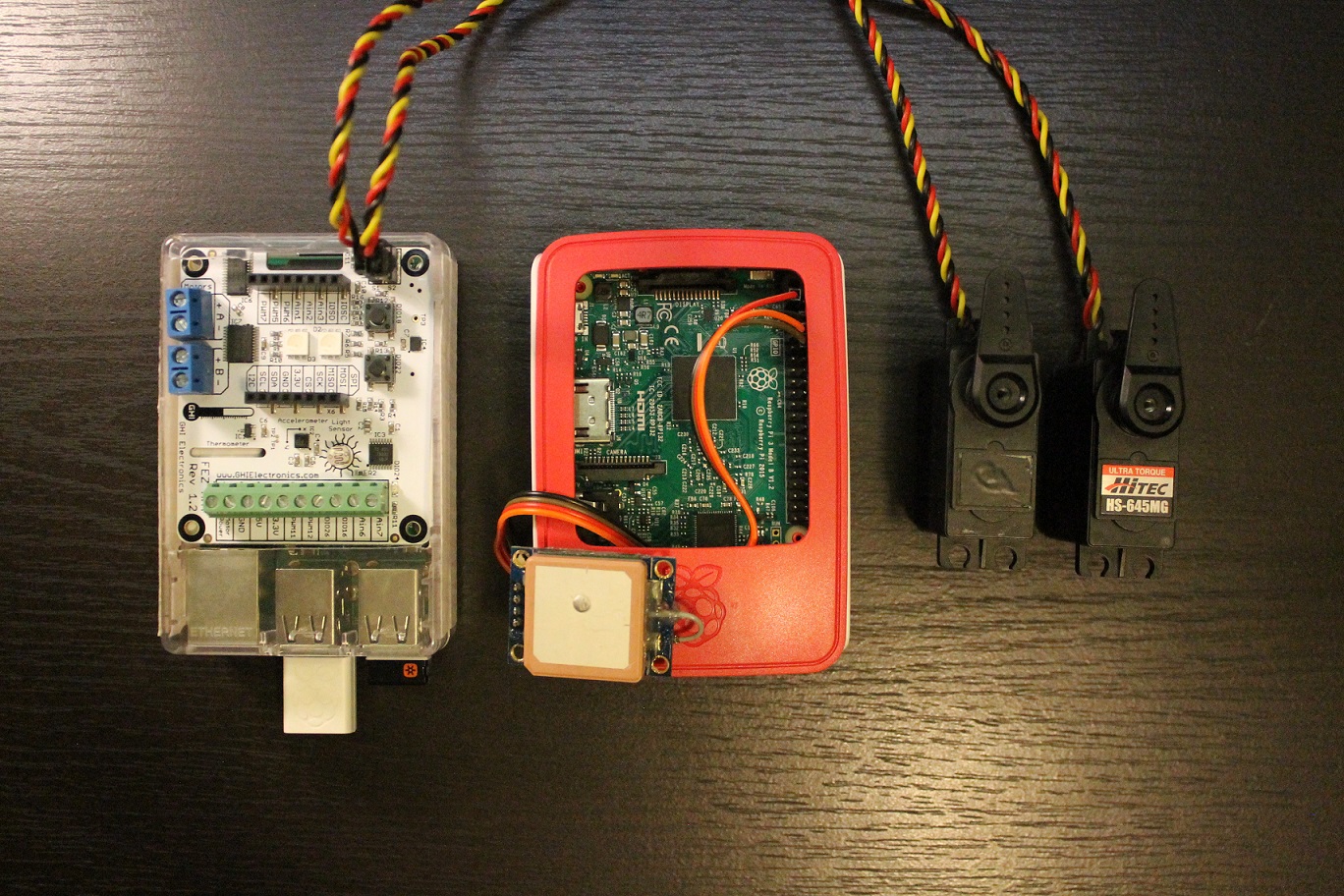
GPS Data Uploader
C#This UWP app is running in Raspberry Pi and it is sending GPS data to the IoT Hub. This particular device uses Andoer NEO-6M GPS. We had to write our own library to parse out the GPS data from its serial interface. We'll be uploading the code soon in our GitHub account.
using System;
using System.Collections.Generic;
//using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using System.Threading.Tasks;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409
namespace SharpGPS
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page
{
// Containment of serial device class
private SerialReceiver serialReceiver;
// Control the whole program with this timer, pulses of GPS data uploaded into IoT Hub
private DispatcherTimer mainTimer;
// GPS NEMA sentence GPGGA data type
GPGGA gpgga;
// GPS data type to be sent as a payload to the IoT Hub
GPSData gpsData;
// Azure IoT Hub instance
IoTHub iotHub;
// Remember this from when we registerd the device
private string iotHubHostName = "";
// Grab these from device details from Azure portal
// ------ WARNING! WARNING! WARNING! ----- Keep these strings somewhere safe in an encrypted configuration file in the production scenarios
// Here it's for illustrative purpose only
private string iotHubDeviceId = "";
private string iotHubDeviceKey = "";
public MainPage()
{
this.InitializeComponent();
// Setup all types simultaneously
SetupSerialReceiver();
SetupGPGGA();
SetupIoTHub();
SetupTimer(30000); // sets up 30 seconds blips, that amounts to about 2880 messages per day * 2 = 5760 messages per day for two devices.
Task.Delay(100000); // Allow GPS to warm up
mainTimer.Start();
mainTimer.Tick += MainTimerOnTick;
}
private async void MainTimerOnTick(object sender,object e)
{
try
{
// Custom formatted GPS data received by parsing the GPGGA sentence
gpsData = gpgga.TryParse(await serialReceiver.ReadAsync(1024));
//Debug.WriteLine(gpsData.ToString());
if(iotHub.IsInitialized)
{
// Upload the GPS data to the IoT Hub as a JSON payload
await iotHub.Upload(gpsData);
}
//Debug.WriteLine("--------------------------------------");
}
catch(Exception ex)
{
throw new SharpGPSException(ex.Message);
}
}
private void SetupIoTHub()
{
iotHub = new IoTHub();
// Pass in the credentials here
iotHub.Initialize(iotHubHostName,iotHubDeviceKey,iotHubDeviceId);
}
private void SetupGPGGA()
{
gpgga = new GPGGA();
gpsData = new GPSData();
}
private async void SetupSerialReceiver()
{
serialReceiver = new SerialReceiver();
await serialReceiver.Initialize();
}
private void SetupTimer(int interval)
{
mainTimer = new DispatcherTimer();
mainTimer.Interval = TimeSpan.FromMilliseconds(interval);
}
}
}
Sensor Data Uploader (Temperature, Light level, Analog In, Servo PWM)
C#This UWP app runs on another Raspberry Pi 2B, and streams sensor data to the same IoT Hub. Stream analytics runs on this data and sends commands back to the Raspberry Pi in form of Events via the Event Hub. Yes! We did it!!
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using System.Threading.Tasks;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=402352&clcid=0x409
namespace SharpActuatorApp
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page
{
private IoTHub iotHub;
private Sensors sensors;
private SensorData sensorData;
private ValueParser valueParser;
private EventHubListener eventHubListener;
// double array to hold the returned values
private double[] returnArray;
private DispatcherTimer mainTimer;
// Ticker field to control the timing of various activities... Thank you: D. Knuth! You, ROCK!
private int ticker = 0;
private string iotHubHostName = "";
private string iotHubDeviceId = "";
private string iotHubDeviceKey = "";
public MainPage()
{
this.InitializeComponent();
SetupIoTHub();
SetupSensors();
SetupValueParser();
SetupSensorData();
SetupEventHubListener();
Task.Delay(60000);
SetupTimer(10000);
mainTimer.Tick += MainTimerOnTick;
}
public async void MainTimerOnTick(object sender, object o)
{
if(eventHubListener.IsInitialized)
{
if(valueParser != null)
{
returnArray = valueParser.TryParse(eventHubListener.Listen());
sensors.ServoControl(returnArray);
Debug.WriteLine("{0},{1}",returnArray[0],returnArray[1]);
}
}
if(ticker == 3)
{
if(sensors.IsInitialized)
{
sensorData = sensors.Read();
if(iotHub.IsInitialized)
{
await iotHub.Upload(sensorData);
Debug.WriteLine(sensorData.ToString());
ticker = 0;
}
}
}
ticker++;
Debug.WriteLine("...");
}
private void SetupTimer(int interval)
{
mainTimer = new DispatcherTimer();
mainTimer.Interval = TimeSpan.FromMilliseconds(interval);
mainTimer.Start();
}
private void SetupValueParser()
{
valueParser = new ValueParser();
}
private async void SetupEventHubListener()
{
eventHubListener = new EventHubListener();
await eventHubListener.Initialize();
}
private void SetupIoTHub()
{
iotHub = new IoTHub();
iotHub.Initialize(iotHubHostName,iotHubDeviceKey,iotHubDeviceId);
}
private void SetupSensors()
{
sensors = new Sensors();
sensors.Initialize();
}
private void SetupSensorData()
{
sensorData = new SensorData();
}
}
}
Thanks to Microsoft .
Comments