Hardware components | ||||||
| × | 1 | ||||
| × | 1 | ||||
| × | 1 | ||||
| × | 2 | ||||
Hand tools and fabrication machines | ||||||
![]() |
| |||||
| ||||||
| ||||||
|
We hate keys. They're the worst.
At FamiLAB, there were a large number of us members with implanted NFC chips in our hands. We decided a good use case for these things was to use it to securely start our motorcycles.
So here we are, ya know... in the future. With a relatively simple circuit, you can hotwire your motorcycle/car/Nintendo 64 to start right up using any sort of NFC tag you might have such as a subway pass, a Disney World card, or even your own embedded hand chip!
Mock Schematic
Head to https://learn.adafruit.com/adafruit-pn532-rfid-nfc/shield-wiring
for updated proper wiring of PN532 shield to Arduino pins.
Pins for Uno (which can be used instead) will be equivalent on Pro Mini.
Pins 11 and 12 on Arduino will be used to Engage and disengage the relay.
I made this to help you see how everything is wired up.
This is the general appearance of the components as they will be wired together. Add an optional on and off switch if you don't ride the motorcycle daily, to conserve power on the motorcycle battery.
for updated proper wiring of PN532 shield to Arduino pins.
Pins for Uno (which can be used instead) will be equivalent on Pro Mini.
Pins 11 and 12 on Arduino will be used to Engage and disengage the relay.
I made this to help you see how everything is wired up.
This is the general appearance of the components as they will be wired together. Add an optional on and off switch if you don't ride the motorcycle daily, to conserve power on the motorcycle battery.
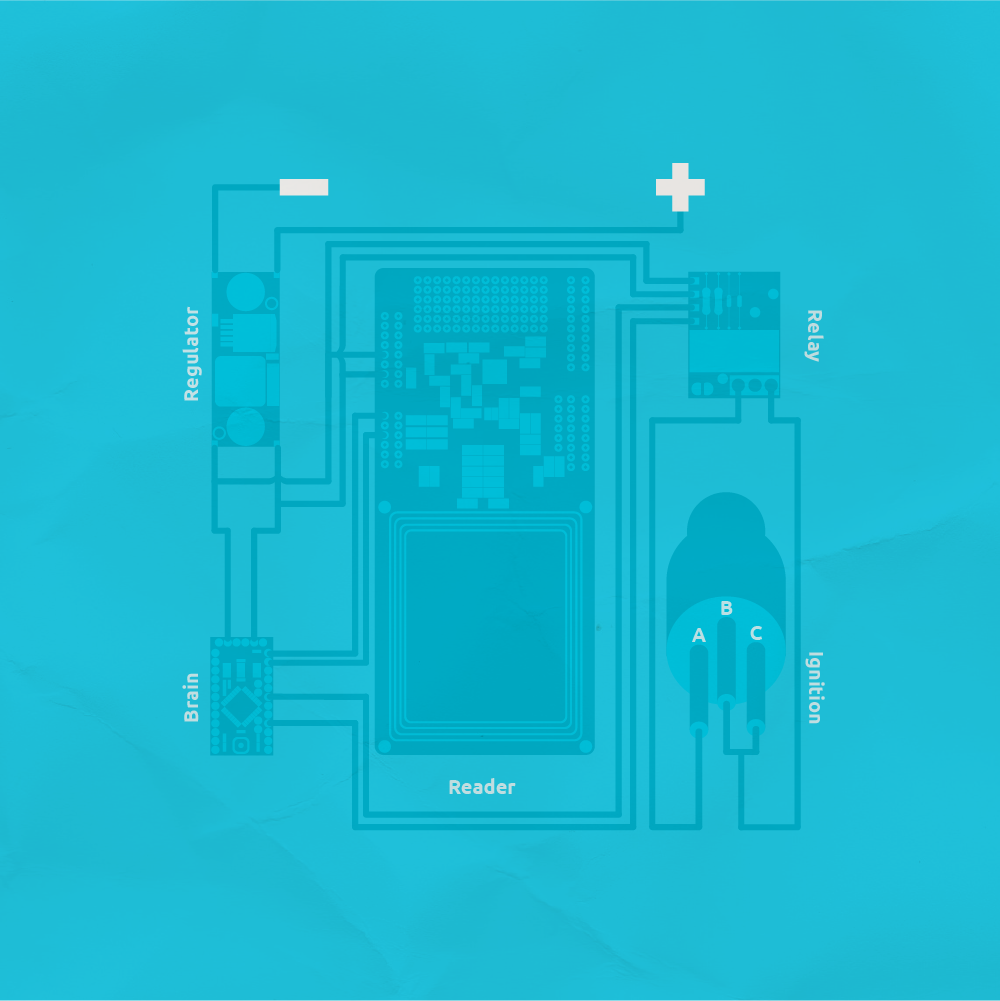
Placement of Reader
I had to make a custom cover for my battery. Harleys are all metal and block the NFC signal. Note: So does carbon fiber.
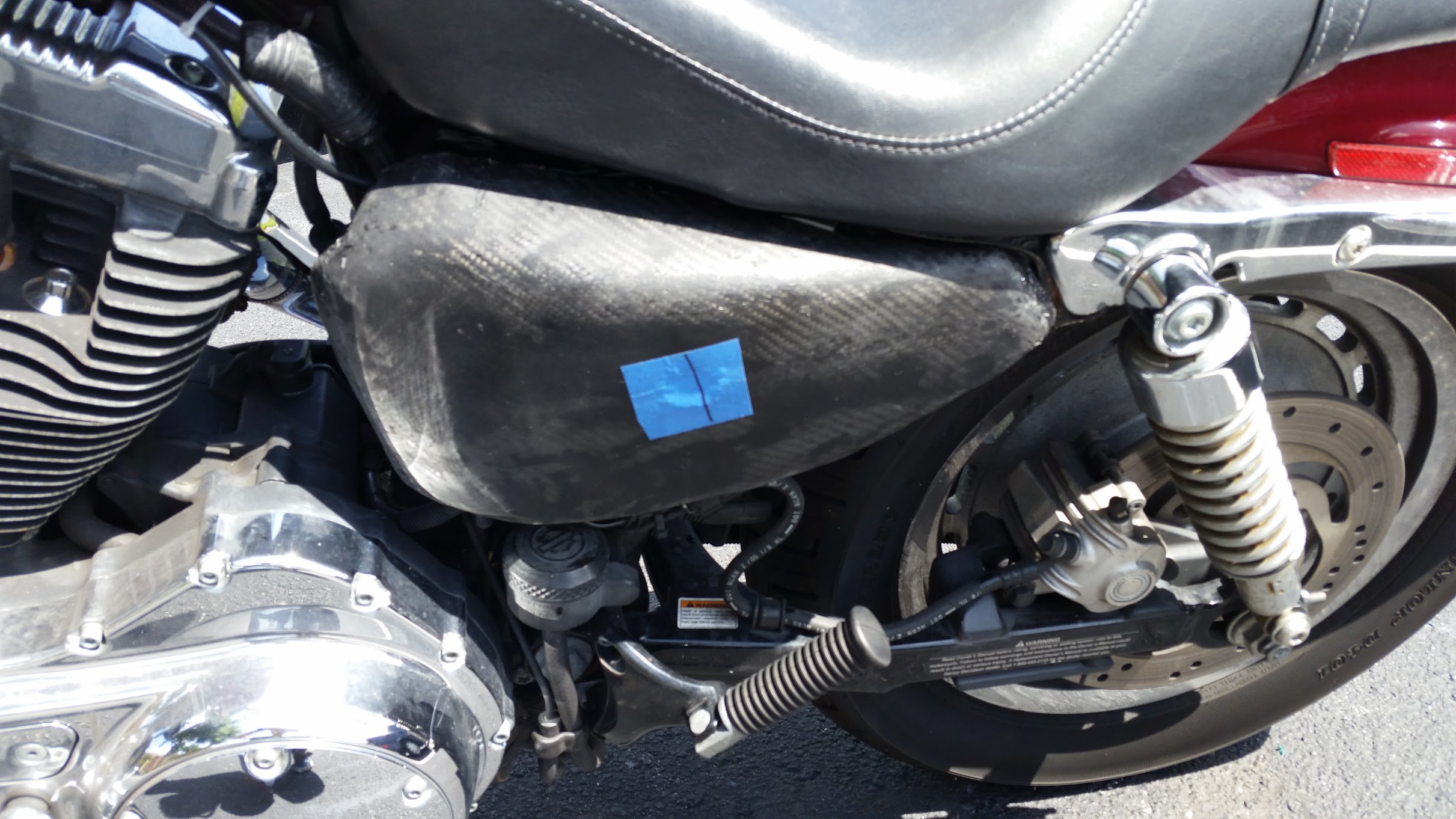
#include <Wire.h>
#include <Adafruit_NFCShield_I2C.h>
#define IRQ (2)
#define RESET (3) // Not connected by default on the NFC Shield
Adafruit_NFCShield_I2C nfc(IRQ, RESET);
void setup(void) {
Serial.begin(9600);
pinMode(13, OUTPUT);
pinMode(12, OUTPUT); // When HIGH, will close relay connection
pinMode(11, OUTPUT); // When HIGH, will open relay connection
nfc.begin();
nfc.SAMConfig();
}
boolean bikeOn = false;
void loop(void) {
//Authorized UIDs
uint8_t auth_uids[][7] = {
// Scan your tag with a phone and place appropriate bytes below
// prefxing each one with "0x" and delimiting with a comma ","
{0x12, 0xFE, 0x12, 0x4E, 0x00, 0x00, 0x00} // Description of Particluar Tag
// ,{0xFE, 0xE2, 0x70, 0x5A, 0x00, 0x00, 0x00} // Add a second one like so
};
uint8_t success;
uint8_t uid[] = { 0, 0, 0, 0, 0, 0, 0 };
uint8_t uidLength;
if (nfc.readPassiveTargetID(PN532_MIFARE_ISO14443A, uid, &uidLength)) {
Serial.print("Entry Request for UID: ");
nfc.PrintHex(uid, uidLength);
uint32_t i;
uint32_t b;
int num_uids = sizeof(auth_uids) / sizeof(auth_uids[0]);
Serial.println(num_uids);
for (i = 0; i < num_uids; i++) {
Serial.print("Comparing UID to: ");
nfc.PrintHex(auth_uids[i], 7);
for (b = 0; b < 7; b++) {
if (uid[b] == auth_uids[i][b]) {
if (b == 6) {
Serial.println("Read and matched!");
if (!bikeOn) {
if (bikeOn) {
Serial.println("bikeOn = True");
}
if (!bikeOn) {
Serial.println("bikeOn = False");
}
bikeOn = true;
if (bikeOn) {
Serial.println("bikeOn = True");
}
if (!bikeOn) {
Serial.println("bikeOn = False");
}
digitalWrite(12, HIGH);
digitalWrite(13, HIGH);
Serial.println("Relay On (Closed/Tripped)");
delay(2000);
digitalWrite(12, LOW);
Serial.println("Delay Done");
} else {
if (bikeOn) {
Serial.println("bikeOn = True");
}
if (!bikeOn) {
Serial.println("bikeOn = False");
}
bikeOn = false;
if (bikeOn) {
Serial.println("bikeOn = True");
}
if (!bikeOn) {
Serial.println("bikeOn = False");
}
digitalWrite(11, HIGH);
digitalWrite(13, LOW);
Serial.println("Relay Off (Open/Untripped)");
delay(2000);
digitalWrite(11, LOW);
Serial.println("Delay Done");
}
i = num_uids;
}
} else {
Serial.println("Failed!");
b = 7;
}
}
}
}
}
You'll need the NFC library from Adafruit, found at https://learn.adafruit.com/adafruit-pn532-rfid-nfc/arduino-library
Instructions for adding a library can be found on Ardunio's website (arduino.cc)
Motorcycles have batteries that output ~12V. We need a regulator to ensure that
the Arduino gets a constant stream of "clean" power so it doesn't shut off
unexpectedly while riding or engaging the starter (in the use case of a
motorcycle). These types of regulators are adjustable. They'll take any voltage
within a certain range and spit out whatever you'd like very reliably. I use a
5V Arduino, but you can use whatever voltage microcontroller you want! This is
where the multimeter comes in.
Latching relays all have slightly different pinouts, but behave generally the same way.
You have power, ground, and two pins to trip the relay to either closed or open.
There are rails to attach the load wire leads.
They should be attached in such a way that if the wires were directly connected
instead of being attached as portrayed in the blue diagram, the device would be
powered on / tripped.
Any relay is usable. Be sure to check the respective data sheet to make sure you don't fry anything.
The following code will accept a specific NFC chip's UUID (sort of like a
computer's MAC address) as a secure means of engaging the relay, in this case
acting like a motorcycle key.
The wiring shown in the diagram is that of a 2005 Harley Davidson and is not
considered a typical ignition circuit. Look up your particular brand and year
to ensure that you rewire it correctly. Don't be afraid. You have fuses, just
in case! Most models should be able to be wired up so that only one relay is
required. I am more than happy to help walk you through any problems you may
have. Just send an email to me@5h4n3.com
Motorcycles often have two different settings for starting. There is an
equivalent to "radio mode" like a car has, where the headlights aren't on, but
you can check the odometer, etc. and there is a full start mode, where you can
hit the starter button and start the bike up.
In this example, there are three pins for the ignition wires (which you should
use thick wires to interface with. The current going through them can be quite
high and will burn through thin wires). If A connects only with B, you get the
radio mode. If both of those are connected and you connect them both to C, the
bike will then think that the key has been turned to the on position and you
will be able to start the bike up.
Shane Engelman
2 projects • 4 followers
Don't call me a programmer. I just hack things together, even if I don't know what I'm doing (which is always the case)
Thanks to Lance Vick and David Sikes.
Comments