Hardware components | ||||||
![]() |
| × | 2 | |||
![]() |
| × | 2 | |||
![]() |
| × | 10 |
It has always been my aspiration to look at a daily product around my life and think how could I improve and build a stronger relationship between it and the users. I chose to increment the desk lamp in this project. This object always sits on our table giving light when we need it to study at night. However, we humans need a break between study from time to time, the usual activity during a break for today’s student is to play social media on a mobile phone. This is time consuming, due to the huge load of information being gained. I have designed this product to make sure that a five- minute break will last five and it will also be a break.
I use two Arduino Mega as a microcontroller for this interactive product. The communication between remote control and the object is possible through Xbee wireless module. The product enclosure was designed in Rhino 3D then export to a laser cutting machine to cut an acrylic sheet. Watch the link to see product in action.
I have also posted this project on PCBWay community, here is the link:
https://www.pcbway.com/project/shareproject/Cubik_Lamp_a9f49b10.html
MORE AWESOME PROJECTS: https://kimbab.me/portfolio/
Cubik Schematic
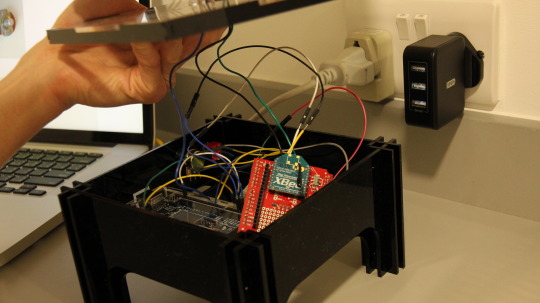
#include <Adafruit_NeoPixel.h>
#define controller Serial1
#define cubik Serial
#define ledPin 3
int incomingByte = 0;
Adafruit_NeoPixel rubikLight = Adafruit_NeoPixel(54, ledPin);
int white[3] = { 238, 150, 238 };
int red[3] = { 255, 0 , 0 };
int blue[3] = { 0 , 0 , 255 };
int violet[3] = { 255, 80 , 0 };
int green[3] = { 0 , 55 , 0 };
int yellow[3] = { 255, 200, 0 };
// int white[3] = { 255, 255, 255 };
// int red[3] = { 255, 0 , 0 };
// int blue[3] = { 0 , 0 , 255 };
// int violet[3] = { 238, 150, 238 };
// int green[3] = { 0 , 55 , 0 };
// int yellow[3] = { 255, 255, 0 };
int colorBufferTH[3] = { 0, 0, 0};
int colorBufferMH[3] = { 0, 0, 0};
int colorBufferBH[3] = { 0, 0, 0};
int colorBufferLVL[3] = { 0, 0, 0};
int colorBufferLVM[3] = { 0, 0, 0};
int colorBufferLVR[3] = { 0, 0, 0};
int colorBufferRVL[3] = { 0, 0, 0};
int colorBufferRVM[3] = { 0, 0, 0};
int colorBufferRVR[3] = { 0, 0, 0};
int topBufferOne[3] = { 0, 0, 0};
int bottomBufferOne[3] = { 0, 0, 0};
int topBufferTwo[3] = { 0, 0, 0};
int bottomBufferTwo[3] = { 0, 0, 0};
int topBufferThree[3] = { 0, 0, 0};
int bottomBufferThree[3] = { 0, 0, 0};
int topBufferFour[3] = { 0, 0, 0};
int bottomBufferFour[3] = { 0, 0, 0};
int topBufferFive[3] = { 0, 0, 0};
int bottomBufferFive[3] = { 0, 0, 0};
int topBufferSix[3] = { 0, 0, 0};
int bottomBufferSix[3] = { 0, 0, 0};
int topBufferSeven[3] = { 0, 0, 0};
int bottomBufferSeven[3] = { 0, 0, 0};
int topBufferEight[3] = { 0, 0, 0};
int bottomBufferEight[3] = { 0, 0, 0};
int leftBufferOne[3] = { 0, 0, 0};
int rightBufferOne[3] = { 0, 0, 0};
int leftBufferTwo[3] = { 0, 0, 0};
int rightBufferTwo[3] = { 0, 0, 0};
int leftBufferThree[3] = { 0, 0, 0};
int rightBufferThree[3] = { 0, 0, 0};
int leftBufferFour[3] = { 0, 0, 0};
int rightBufferFour[3] = { 0, 0, 0};
int leftBufferFive[3] = { 0, 0, 0};
int rightBufferFive[3] = { 0, 0, 0};
int leftBufferSix[3] = { 0, 0, 0};
int rightBufferSix[3] = { 0, 0, 0};
int leftBufferSeven[3] = { 0, 0, 0};
int rightBufferSeven[3] = { 0, 0, 0};
int leftBufferEight[3] = { 0, 0, 0};
int rightBufferEight[3] = { 0, 0, 0};
int frontBufferOne[3] = { 0, 0, 0};
int backBufferOne[3] = { 0, 0, 0};
int frontBufferTwo[3] = { 0, 0, 0};
int backBufferTwo[3] = { 0, 0, 0};
int frontBufferThree[3] = { 0, 0, 0};
int backBufferThree[3] = { 0, 0, 0};
int frontBufferFour[3] = { 0, 0, 0};
int backBufferFour[3] = { 0, 0, 0};
int frontBufferFive[3] = { 0, 0, 0};
int backBufferFive[3] = { 0, 0, 0};
int frontBufferSix[3] = { 0, 0, 0};
int backBufferSix[3] = { 0, 0, 0};
int frontBufferSeven[3] = { 0, 0, 0};
int backBufferSeven[3] = { 0, 0, 0};
int frontBufferEight[3] = { 0, 0, 0};
int backBufferEight[3] = { 0, 0, 0};
int FirstPanel[3][3][4] = { {{0 , red[0] , red[1] , red[2] } , {1 , red[0] , red[1] , red[2] } , {2 , red[0] , red[1] , red[2] }} , {{3 , red[0] , red[1] , red[2] } , {4 , red[0] , red[1] , red[2] } , {5 , red[0] , red[1] , red[2] }} , {{6 , red[0] , red[1] , red[2] } , {7 , red[0] , red[1] , red[2] } , {8 , red[0] , red[1] , red[2] }} };
int SecondPanel[3][3][4] = { {{9 , green[0] , green[1] , green[2] } , {10, green[0] , green[1] , green[2] } , {11, green[0] , green[1] , green[2] }} , {{12, green[0] , green[1] , green[2] } , {13, green[0] , green[1] , green[2] } , {14, green[0] , green[1] , green[2] }} , {{15, green[0] , green[1] , green[2] } , {16, green[0] , green[1] , green[2] } , {17, green[0] , green[1] , green[2] }} };
int ThirdPanel[3][3][4] = { {{18, violet[0], violet[1], violet[2]} , {19, violet[0], violet[1], violet[2]} , {20, violet[0], violet[1], violet[2]}} , {{21, violet[0], violet[1], violet[2]} , {22, violet[0], violet[1], violet[2]} , {23, violet[0], violet[1], violet[2]}} , {{24, violet[0], violet[1], violet[2]} , {25, violet[0], violet[1], violet[2]} , {26, violet[0], violet[1], violet[2]}} };
int FourthPanel[3][3][4] = { {{27, blue[0] , blue[1] , blue[2] } , {28, blue[0] , blue[1] , blue[2] } , {29, blue[0] , blue[1] , blue[2] }} , {{30, blue[0] , blue[1] , blue[2] } , {31, blue[0] , blue[1] , blue[2] } , {32, blue[0] , blue[1] , blue[2] }} , {{33, blue[0] , blue[1] , blue[2] } , {34, blue[0] , blue[1] , blue[2] } , {35, blue[0] , blue[1] , blue[2] }} };
int FifthPanel[3][3][4] = { {{36, white[0] , white[1] , white[2] } , {37, white[0] , white[1] , white[2] } , {38, white[0] , white[1] , white[2] }} , {{39, white[0] , white[1] , white[2] } , {40, white[0] , white[1] , white[2] } , {41, white[0] , white[1] , white[2] }} , {{42, white[0] , white[1] , white[2] } , {43, white[0] , white[1] , white[2] } , {44, white[0] , white[1] , white[2] }} };
int SixPanel[3][3][4] = { {{45, yellow[0], yellow[1], yellow[2]} , {46, yellow[0], yellow[1], yellow[2]} , {47, yellow[0], yellow[1], yellow[2]}} , {{48, yellow[0], yellow[1], yellow[2]} , {49, yellow[0], yellow[1], yellow[2]} , {50, yellow[0], yellow[1], yellow[2]}} , {{51, yellow[0], yellow[1], yellow[2]} , {52, yellow[0], yellow[1], yellow[2]} , {53, yellow[0], yellow[1], yellow[2]}} };
//Horizontal movement
int horizontalFirst[3][3] = {{0,1,2} , {3,4,5} , {6,7,8} };
int horizontalSecond[3][3] = {{9,10,11} , {12,13,14} , {15,16,17}};
int horizontalThird[3][3] = {{18,19,20} , {21,22,23} , {24,25,26}};
int horizontalFourth[3][3] = {{27,28,29} , {30,31,32} , {33,34,35}};
//left vertical movement
int leftVerticalFirst[3][3] = {{2,5,8} , {1,4,7} , {0,3,6} };
int leftVerticalFifth[3][3] = {{38,41,44} , {37,40,43} , {36,39,42}};
int leftVerticalThird[3][3] = {{24,21,18} , {25,22,19} , {26,23,20}};
int leftVerticalSix[3][3] = {{47,50,53} , {46,49,52} , {45,48,51}};
//right vertical movement
int rightVerticalFourth[3][3] = {{29,32,35} , {28,31,34} , {27,30,33}};
int rightVerticalFifth[3][3] = {{44,43,42} , {41,40,39} , {38,37,36}};
int rightVerticalSix[3][3] = {{45,46,47} , {48,49,50} , {51,52,53}};
int rightVerticalSecond[3][3] = {{15,12,9} , {16,13,10} , {17,14,11}};
int incoming;
int tl, currentTL;
int tm, currentTM;
int tr, currentTR;
int ml, currentML;
int mm, currentMM;
int mr, currentMR;
int bl, currentBL;
int bm, currentBM;
int br, currentBR;
int lampSwitch = 2;
int pot = A0;
int colorVal;
int sensVal;
int rotVal;
int curRotVal;
int rotValTwo;
int curRotValTwo;
unsigned long time;
void setup() {
rubikLight.begin();
rubikLight.show();
// put your setup code here, to run once:
controller.begin(9600);
cubik.begin(9600);
pinMode(lampSwitch , INPUT);
pinMode(pot , INPUT);
pinMode(ledPin , OUTPUT);
curRotVal = analogRead(pot);
rubikLight.setBrightness(255);
currentTL = 0;
currentTM = 0;
currentTR = 0;
currentML = 0;
currentMM = 0;
currentMR = 0;
currentBL = 0;
currentBM = 0;
currentBR = 0;
}
void loop() {
// put your main code here, to run repeatedly:
if (digitalRead(lampSwitch) == HIGH) {
colorVal = map(analogRead(pot),0,1024,0,255);
for(int i = 0 ; i < rubikLight.numPixels() ; i++) {
rubikLight.setPixelColor(i,0,0,colorVal);
}
rubikLight.show();
} else if (digitalRead(lampSwitch) == LOW) {
for (int row = 0; row < 3; row++)
{
for (int col = 0; col < 3; col++)
{
rubikLight.setPixelColor(FirstPanel[row][col][0], FirstPanel[row][col][1], FirstPanel[row][col][2], FirstPanel[row][col][3]);
rubikLight.setPixelColor(SecondPanel[row][col][0], SecondPanel[row][col][1], SecondPanel[row][col][2], SecondPanel[row][col][3]);
rubikLight.setPixelColor(ThirdPanel[row][col][0], ThirdPanel[row][col][1], ThirdPanel[row][col][2], ThirdPanel[row][col][3]);
rubikLight.setPixelColor(FourthPanel[row][col][0], FourthPanel[row][col][1], FourthPanel[row][col][2], FourthPanel[row][col][3]);
rubikLight.setPixelColor(FifthPanel[row][col][0], FifthPanel[row][col][1], FifthPanel[row][col][2], FifthPanel[row][col][3]);
rubikLight.setPixelColor(SixPanel[row][col][0], SixPanel[row][col][1], SixPanel[row][col][2], SixPanel[row][col][3]);
}
}
rubikLight.show();
if(controller.available() > 0) {
incoming = controller.read();
//cubik.write(incoming);
if(incoming == 'A') {
tl = controller.parseInt();
cubik.print("TL ");
cubik.println(tl);
}
if(incoming == 'B') {
tm = controller.parseInt();
cubik.print("TM ");
cubik.println(tm);
}
if(incoming == 'C') {
tr = controller.parseInt();
cubik.print("TR ");
cubik.println(tr);
}
if(incoming == 'D') {
ml = controller.parseInt();
cubik.print("ML ");
cubik.println(ml);
}
if(incoming == 'E') {
mm = controller.parseInt();
cubik.print("MM ");
cubik.println(mm);
}
if(incoming == 'F') {
mr = controller.parseInt();
cubik.print("MR ");
cubik.println(mr);
}
if(incoming == 'G') {
bl = controller.parseInt();
cubik.print("BL ");
cubik.println(bl);
}
if(incoming == 'H') {
bm = controller.parseInt();
cubik.print("BM ");
cubik.println(bm);
}
if(incoming == 'T') {
br = controller.parseInt();
cubik.print("BR ");
cubik.println(br);
}
//---------------------------------------------------------------------------------------------------------------------------------------------
// Horizontal sides move
//---------------------------------------------------------------------------------------------------------------------------------------------
//rotate leds top horizontal line
if( currentTL - tl >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
if ( horizontalFirst[0][k] == FirstPanel[i][j][0] ) {
colorBufferTH[0] = FourthPanel[i][j][1];
colorBufferTH[1] = FourthPanel[i][j][2];
colorBufferTH[2] = FourthPanel[i][j][3];
FourthPanel[i][j][1] = FirstPanel[i][j][1];
FourthPanel[i][j][2] = FirstPanel[i][j][2];
FourthPanel[i][j][3] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = SecondPanel[i][j][1];
FirstPanel[i][j][2] = SecondPanel[i][j][2];
FirstPanel[i][j][3] = SecondPanel[i][j][3];
SecondPanel[i][j][1] = ThirdPanel[i][j][1];
SecondPanel[i][j][2] = ThirdPanel[i][j][2];
SecondPanel[i][j][3] = ThirdPanel[i][j][3];
ThirdPanel[i][j][1] = colorBufferTH[0];
ThirdPanel[i][j][2] = colorBufferTH[1];
ThirdPanel[i][j][3] = colorBufferTH[2];
}
}
}
}
//move right
for (int i = 0; i < 3; ++i)
{
//36
topBufferOne[i] = FifthPanel[0][0][i + 1];
//37
topBufferTwo[i] = FifthPanel[0][1][i + 1];
//38
topBufferThree[i] = FifthPanel[0][2][i + 1];
//39
topBufferFour[i] = FifthPanel[1][0][i + 1];
//41
topBufferFive[i] = FifthPanel[1][2][i + 1];
//42
topBufferSix[i] = FifthPanel[2][0][i + 1];
//43
topBufferSeven[i] = FifthPanel[2][1][i + 1];
//44
topBufferEight[i] = FifthPanel[2][2][i + 1];
//first row 36 to 42
FifthPanel[0][0][i + 1] = topBufferSix[i];
// 37 to 39
FifthPanel[0][1][i + 1] = topBufferFour[i];
// 38 to 36
FifthPanel[0][2][i + 1] = topBufferOne[i];
//second row 39 to 43
FifthPanel[1][0][i + 1] = topBufferSeven[i];
// 41 to 37
FifthPanel[1][2][i + 1] = topBufferTwo[i];
//third row 42 to 44
FifthPanel[2][0][i + 1] = topBufferEight[i];
// 43 to 41
FifthPanel[2][1][i + 1] = topBufferFive[i];
// 44 to 38
FifthPanel[2][2][i + 1] = topBufferThree[i];
}
currentTL = tl;
cubik.print("CurrentTL ");
cubik.println(currentTL);
} else if (tl - currentTL >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
if ( horizontalFirst[0][k] == FirstPanel[i][j][0] ) {
colorBufferTH[0] = FourthPanel[i][j][1];
colorBufferTH[1] = FourthPanel[i][j][2];
colorBufferTH[2] = FourthPanel[i][j][3];
FourthPanel[i][j][1] = ThirdPanel[i][j][1];
FourthPanel[i][j][2] = ThirdPanel[i][j][2];
FourthPanel[i][j][3] = ThirdPanel[i][j][3];
ThirdPanel[i][j][1] = SecondPanel[i][j][1];
ThirdPanel[i][j][2] = SecondPanel[i][j][2];
ThirdPanel[i][j][3] = SecondPanel[i][j][3];
SecondPanel[i][j][1] = FirstPanel[i][j][1];
SecondPanel[i][j][2] = FirstPanel[i][j][2];
SecondPanel[i][j][3] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = colorBufferTH[0];
FirstPanel[i][j][2] = colorBufferTH[1];
FirstPanel[i][j][3] = colorBufferTH[2];
}
}
}
}
//move left
for (int i = 0; i < 3; ++i)
{
//36
topBufferOne[i] = FifthPanel[0][0][i + 1];
//37
topBufferTwo[i] = FifthPanel[0][1][i + 1];
//38
topBufferThree[i] = FifthPanel[0][2][i + 1];
//39
topBufferFour[i] = FifthPanel[1][0][i + 1];
//41
topBufferFive[i] = FifthPanel[1][2][i + 1];
//42
topBufferSix[i] = FifthPanel[2][0][i + 1];
//43
topBufferSeven[i] = FifthPanel[2][1][i + 1];
//44
topBufferEight[i] = FifthPanel[2][2][i + 1];
//first row 36 to 38
FifthPanel[0][0][i + 1] = topBufferThree[i];
// 37 to 41
FifthPanel[0][1][i + 1] = topBufferFive[i];
// 38 to 44
FifthPanel[0][2][i + 1] = topBufferEight[i];
//second row 39 to 37
FifthPanel[1][0][i + 1] = topBufferTwo[i];
// 41 to 43
FifthPanel[1][2][i + 1] = topBufferSeven[i];
//third row 42 to 36
FifthPanel[2][0][i + 1] = topBufferOne[i];
// 43 to 39
FifthPanel[2][1][i + 1] = topBufferFour[i];
// 44 to 42
FifthPanel[2][2][i + 1] = topBufferSix[i];
}
currentTL = tl;
cubik.print("CurrentTL ");
cubik.println(currentTL);
}
//---------------------------------------------------------------------------------------------------------------------------------------------
//rotate leds middle horizontal line
if( currentTM - tm >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
if ( horizontalFirst[1][k] == FirstPanel[i][j][0] ) {
colorBufferMH[0] = FourthPanel[i][j][1];
colorBufferMH[1] = FourthPanel[i][j][2];
colorBufferMH[2] = FourthPanel[i][j][3];
FourthPanel[i][j][1] = FirstPanel[i][j][1];
FourthPanel[i][j][2] = FirstPanel[i][j][2];
FourthPanel[i][j][3] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = SecondPanel[i][j][1];
FirstPanel[i][j][2] = SecondPanel[i][j][2];
FirstPanel[i][j][3] = SecondPanel[i][j][3];
SecondPanel[i][j][1] = ThirdPanel[i][j][1];
SecondPanel[i][j][2] = ThirdPanel[i][j][2];
SecondPanel[i][j][3] = ThirdPanel[i][j][3];
ThirdPanel[i][j][1] = colorBufferMH[0];
ThirdPanel[i][j][2] = colorBufferMH[1];
ThirdPanel[i][j][3] = colorBufferMH[2];
}
}
}
}
currentTM = tm;
cubik.print("CurrentTM ");
cubik.println(currentTM);
} else if (tm - currentTM >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
if ( horizontalFirst[1][k] == FirstPanel[i][j][0] ) {
colorBufferMH[0] = FourthPanel[i][j][1];
colorBufferMH[1] = FourthPanel[i][j][2];
colorBufferMH[2] = FourthPanel[i][j][3];
FourthPanel[i][j][1] = ThirdPanel[i][j][1];
FourthPanel[i][j][2] = ThirdPanel[i][j][2];
FourthPanel[i][j][3] = ThirdPanel[i][j][3];
ThirdPanel[i][j][1] = SecondPanel[i][j][1];
ThirdPanel[i][j][2] = SecondPanel[i][j][2];
ThirdPanel[i][j][3] = SecondPanel[i][j][3];
SecondPanel[i][j][1] = FirstPanel[i][j][1];
SecondPanel[i][j][2] = FirstPanel[i][j][2];
SecondPanel[i][j][3] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = colorBufferMH[0];
FirstPanel[i][j][2] = colorBufferMH[1];
FirstPanel[i][j][3] = colorBufferMH[2];
}
}
}
}
currentTM = tm;
cubik.print("CurrentTM ");
cubik.println(currentTM);
}
//---------------------------------------------------------------------------------------------------------------------------------------------
//rotate leds bottom horizontal line
if( currentTR - tr >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
if ( horizontalFirst[2][k] == FirstPanel[i][j][0] ) {
colorBufferBH[0] = FourthPanel[i][j][1];
colorBufferBH[1] = FourthPanel[i][j][2];
colorBufferBH[2] = FourthPanel[i][j][3];
FourthPanel[i][j][1] = FirstPanel[i][j][1];
FourthPanel[i][j][2] = FirstPanel[i][j][2];
FourthPanel[i][j][3] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = SecondPanel[i][j][1];
FirstPanel[i][j][2] = SecondPanel[i][j][2];
FirstPanel[i][j][3] = SecondPanel[i][j][3];
SecondPanel[i][j][1] = ThirdPanel[i][j][1];
SecondPanel[i][j][2] = ThirdPanel[i][j][2];
SecondPanel[i][j][3] = ThirdPanel[i][j][3];
ThirdPanel[i][j][1] = colorBufferBH[0];
ThirdPanel[i][j][2] = colorBufferBH[1];
ThirdPanel[i][j][3] = colorBufferBH[2];
}
}
}
}
//move right
for (int i = 0; i < 3; ++i)
{
//45
bottomBufferOne[i] = SixPanel[0][0][i + 1];
//46
bottomBufferTwo[i] = SixPanel[0][1][i + 1];
//47
bottomBufferThree[i] = SixPanel[0][2][i + 1];
//48
bottomBufferFour[i] = SixPanel[1][0][i + 1];
//50
bottomBufferFive[i] = SixPanel[1][2][i + 1];
//51
bottomBufferSix[i] = SixPanel[2][0][i + 1];
//52
bottomBufferSeven[i] = SixPanel[2][1][i + 1];
//53
bottomBufferEight[i] = SixPanel[2][2][i + 1];
//first row 45 to 47
SixPanel[0][0][i + 1] = bottomBufferThree[i];
// 46 to 50
SixPanel[0][1][i + 1] = bottomBufferFive[i];
// 47 to 53
SixPanel[0][2][i + 1] = bottomBufferEight[i];
//second row 48 to 46
SixPanel[1][0][i + 1] = bottomBufferTwo[i];
// 50 to 52
SixPanel[1][2][i + 1] = bottomBufferSeven[i];
//third row 51 to 45
SixPanel[2][0][i + 1] = bottomBufferOne[i];
// 52 to 48
SixPanel[2][1][i + 1] = bottomBufferFour[i];
// 53 to 51
SixPanel[2][2][i + 1] = bottomBufferSix[i];
}
currentTR = tr;
cubik.print("CurrentTR ");
cubik.println(currentTR);
} else if (tr - currentTR >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
if ( horizontalFirst[2][k] == FirstPanel[i][j][0] ) {
colorBufferBH[0] = FourthPanel[i][j][1];
colorBufferBH[1] = FourthPanel[i][j][2];
colorBufferBH[2] = FourthPanel[i][j][3];
FourthPanel[i][j][1] = ThirdPanel[i][j][1];
FourthPanel[i][j][2] = ThirdPanel[i][j][2];
FourthPanel[i][j][3] = ThirdPanel[i][j][3];
ThirdPanel[i][j][1] = SecondPanel[i][j][1];
ThirdPanel[i][j][2] = SecondPanel[i][j][2];
ThirdPanel[i][j][3] = SecondPanel[i][j][3];
SecondPanel[i][j][1] = FirstPanel[i][j][1];
SecondPanel[i][j][2] = FirstPanel[i][j][2];
SecondPanel[i][j][3] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = colorBufferBH[0];
FirstPanel[i][j][2] = colorBufferBH[1];
FirstPanel[i][j][3] = colorBufferBH[2];
}
}
}
}
//move left
for (int i = 0; i < 3; ++i)
{
//45
bottomBufferOne[i] = SixPanel[0][0][i + 1];
//46
bottomBufferTwo[i] = SixPanel[0][1][i + 1];
//47
bottomBufferThree[i] = SixPanel[0][2][i + 1];
//48
bottomBufferFour[i] = SixPanel[1][0][i + 1];
//50
bottomBufferFive[i] = SixPanel[1][2][i + 1];
//51
bottomBufferSix[i] = SixPanel[2][0][i + 1];
//52
bottomBufferSeven[i] = SixPanel[2][1][i + 1];
//53
bottomBufferEight[i] = SixPanel[2][2][i + 1];
//first row 45 to 51
SixPanel[0][0][i + 1] = bottomBufferSix[i];
// 46 to 48
SixPanel[0][1][i + 1] = bottomBufferFour[i];
// 47 to 45
SixPanel[0][2][i + 1] = bottomBufferOne[i];
//second row 48 to 50
SixPanel[1][0][i + 1] = bottomBufferFive[i];
// 50 to 46
SixPanel[1][2][i + 1] = bottomBufferTwo[i];
//third row 51 to 53
SixPanel[2][0][i + 1] = bottomBufferEight[i];
// 52 to 46
SixPanel[2][1][i + 1] = bottomBufferTwo[i];
// 53 to 47
SixPanel[2][2][i + 1] = bottomBufferThree[i];
}
currentTR = tr;
cubik.print("CurrentTR ");
cubik.println(currentTR);
}
//---------------------------------------------------------------------------------------------------------------------------------------------
// Left Vertical
//---------------------------------------------------------------------------------------------------------------------------------------------
//rotate lEDs left vertical left line
if( currentMR - mr >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
for (int l = 0; l < 3; l++)
{
for (int m = 0; m < 3; m++)
{
if ( leftVerticalFirst[0][k] == FirstPanel[i][j][0] && leftVerticalThird[0][k] == ThirdPanel[l][m][0]) {
colorBufferLVL[0] = FirstPanel[i][j][1];
colorBufferLVL[1] = FirstPanel[i][j][2];
colorBufferLVL[2] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = SixPanel[i][j][1];
FirstPanel[i][j][2] = SixPanel[i][j][2];
FirstPanel[i][j][3] = SixPanel[i][j][3];
SixPanel[i][j][1] = ThirdPanel[l][m][1];
SixPanel[i][j][2] = ThirdPanel[l][m][2];
SixPanel[i][j][3] = ThirdPanel[l][m][3];
ThirdPanel[l][m][1] = FifthPanel[i][j][1];
ThirdPanel[l][m][2] = FifthPanel[i][j][2];
ThirdPanel[l][m][3] = FifthPanel[i][j][3];
FifthPanel[i][j][1] = colorBufferLVL[0];
FifthPanel[i][j][2] = colorBufferLVL[1];
FifthPanel[i][j][3] = colorBufferLVL[2];
}
}
}
}
}
}
//move right
for (int i = 0; i < 3; ++i)
{
//9
leftBufferOne[i] = SecondPanel[0][0][i + 1];
//10
leftBufferTwo[i] = SecondPanel[0][1][i + 1];
//11
leftBufferThree[i] = SecondPanel[0][2][i + 1];
//12
leftBufferFour[i] = SecondPanel[1][0][i + 1];
//14
leftBufferFive[i] = SecondPanel[1][2][i + 1];
//15
leftBufferSix[i] = SecondPanel[2][0][i + 1];
//16
leftBufferSeven[i] = SecondPanel[2][1][i + 1];
//17
leftBufferEight[i] = SecondPanel[2][2][i + 1];
//first row 9 to 15
SecondPanel[0][0][i + 1] = leftBufferSix[i];
// 10 to 12
SecondPanel[0][1][i + 1] = leftBufferFour[i];
// 11 to 9
SecondPanel[0][2][i + 1] = leftBufferOne[i];
//second row 12 to 16
SecondPanel[1][0][i + 1] = leftBufferSeven[i];
// 14 to 10
SecondPanel[1][2][i + 1] = leftBufferTwo[i];
//third row 15 to 17
SecondPanel[2][0][i + 1] = leftBufferEight[i];
// 16 to 14
SecondPanel[2][1][i + 1] = leftBufferFive[i];
// 17 to 11
SecondPanel[2][2][i + 1] = leftBufferThree[i];
}
currentMR = mr;
cubik.print("CurrentMR ");
cubik.println(currentMR);
} else if ( mr - currentMR >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
for (int l = 0; l < 3; l++)
{
for (int m = 0; m < 3; m++)
{
if ( leftVerticalFirst[0][k] == FirstPanel[i][j][0] && leftVerticalThird[0][k] == ThirdPanel[l][m][0]) {
colorBufferLVL[0] = FirstPanel[i][j][1];
colorBufferLVL[1] = FirstPanel[i][j][2];
colorBufferLVL[2] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = FifthPanel[i][j][1];
FirstPanel[i][j][2] = FifthPanel[i][j][2];
FirstPanel[i][j][3] = FifthPanel[i][j][3];
FifthPanel[i][j][1] = ThirdPanel[l][m][1];
FifthPanel[i][j][2] = ThirdPanel[l][m][2];
FifthPanel[i][j][3] = ThirdPanel[l][m][3];
ThirdPanel[l][m][1] = SixPanel[i][j][1];
ThirdPanel[l][m][2] = SixPanel[i][j][2];
ThirdPanel[l][m][3] = SixPanel[i][j][3];
SixPanel[i][j][1] = colorBufferLVL[0];
SixPanel[i][j][2] = colorBufferLVL[1];
SixPanel[i][j][3] = colorBufferLVL[2];
}
}
}
}
}
}
//move left
for (int i = 0; i < 3; ++i)
{
//9
leftBufferOne[i] = SecondPanel[0][0][i + 1];
//10
leftBufferTwo[i] = SecondPanel[0][1][i + 1];
//11
leftBufferThree[i] = SecondPanel[0][2][i + 1];
//12
leftBufferFour[i] = SecondPanel[1][0][i + 1];
//14
leftBufferFive[i] = SecondPanel[1][2][i + 1];
//15
leftBufferSix[i] = SecondPanel[2][0][i + 1];
//16
leftBufferSeven[i] = SecondPanel[2][1][i + 1];
//17
leftBufferEight[i] = SecondPanel[2][2][i + 1];
//first row 9 to 11
SecondPanel[0][0][i + 1] = leftBufferThree[i];
// 10 to 14
SecondPanel[0][1][i + 1] = leftBufferFive[i];
// 11 to 17
SecondPanel[0][2][i + 1] = leftBufferEight[i];
//second row 12 to 10
SecondPanel[1][0][i + 1] = leftBufferTwo[i];
// 14 to 16
SecondPanel[1][2][i + 1] = leftBufferSeven[i];
//third row 15 to 9
SecondPanel[2][0][i + 1] = leftBufferOne[i];
// 16 to 12
SecondPanel[2][1][i + 1] = leftBufferFour[i];
// 17 to 15
SecondPanel[2][2][i + 1] = leftBufferSix[i];
}
currentMR = mr;
cubik.print("CurrentMR ");
cubik.println(currentMR);
}
//---------------------------------------------------------------------------------------------------------------------------------------------
//rotate lEDs left vertical middle line
if( currentMM - mm >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
for (int l = 0; l < 3; l++)
{
for (int m = 0; m < 3; m++)
{
if ( leftVerticalFirst[1][k] == FirstPanel[i][j][0] && leftVerticalThird[1][k] == ThirdPanel[l][m][0]) {
colorBufferLVM[0] = FirstPanel[i][j][1];
colorBufferLVM[1] = FirstPanel[i][j][2];
colorBufferLVM[2] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = SixPanel[i][j][1];
FirstPanel[i][j][2] = SixPanel[i][j][2];
FirstPanel[i][j][3] = SixPanel[i][j][3];
SixPanel[i][j][1] = ThirdPanel[l][m][1];
SixPanel[i][j][2] = ThirdPanel[l][m][2];
SixPanel[i][j][3] = ThirdPanel[l][m][3];
ThirdPanel[l][m][1] = FifthPanel[i][j][1];
ThirdPanel[l][m][2] = FifthPanel[i][j][2];
ThirdPanel[l][m][3] = FifthPanel[i][j][3];
FifthPanel[i][j][1] = colorBufferLVM[0];
FifthPanel[i][j][2] = colorBufferLVM[1];
FifthPanel[i][j][3] = colorBufferLVM[2];
}
}
}
}
}
}
currentMM = mm;
cubik.print("CurrentMM ");
cubik.println(currentMM);
} else if ( mm - currentMM >= 2) {
for (int k = 0; k < 3; k++)
{
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
for (int l = 0; l < 3; l++)
{
for (int m = 0; m < 3; m++)
{
if ( leftVerticalFirst[1][k] == FirstPanel[i][j][0] && leftVerticalThird[1][k] == ThirdPanel[l][m][0]) {
colorBufferLVM[0] = FirstPanel[i][j][1];
colorBufferLVM[1] = FirstPanel[i][j][2];
colorBufferLVM[2] = FirstPanel[i][j][3];
FirstPanel[i][j][1] = FifthPanel[i][j][1];
FirstPanel[i][j][2] = FifthPanel[i][j][2];
FirstPanel[i][j][3] = FifthPanel[i][j][3];
FifthPanel[i][j][1] = ThirdPanel[l][m][1];
FifthPanel[i][j][2] = ThirdPanel[l][m][2];
FifthPanel[i][j][3] = ThirdPanel[l][m][3];
ThirdPanel[l][m][1] = SixPanel[i][j][1];
ThirdPanel[l][m][2] = SixPanel[i][j][2];
ThirdPanel[l][m][3] = SixPanel[i][j][3];
SixPanel[i][j][1] = colorBufferLVM[0];
SixPanel[i][j][2] = colorBufferLVM[1];
SixPanel[i][j][3] = colorBufferLVM[2];
}
}
}
}
}
}
currentMM = mm;
cubik.print("CurrentMM ");
cubik.println(currentMM);
}
...
This file has been truncated, please download it to see its full contents.
Comments