Hardware components | ||||||
![]() |
| × | 1 | |||
![]() |
| × | 1 | |||
![]() |
| × | 2 | |||
![]() |
| × | 2 | |||
![]() |
| × | 3 | |||
![]() |
| × | 3 | |||
| × | 1 | ||||
![]() |
| × | 10 |
Lobot was the mute cyborg that served as the primary interface to all of Cloud City's systems. He was capable of communicating with the city's central computer and manipulate things such as security, repulsor lifts, communication and life support systems.
The Lobot Simulator will work in the same vein as the cyborg with a few twists. I've implemented espeak functionality so the system provides exact updates for what it is doing. The primary interface will be a DJango (https://www.djangoproject.com/) web app that I can control from my tablet or phone. I created a simple Photon circuit that is connected to a series of multi-color LEDs. These LEDs will light up depending on which command is chosen from the interface. The simulator will have the following features to somewhat replicate what Lobot does:
- Repulsor Lift --> Robotic Arm Control (Green LED Lights)
- Security --> Security Camera Manipulation (Red LED Lights)
- Communications --> SMS Messaging (Blue LED Lights)
- Life Support --> Telephony simulation (Gold LED Lights)
BrickPi Illustration
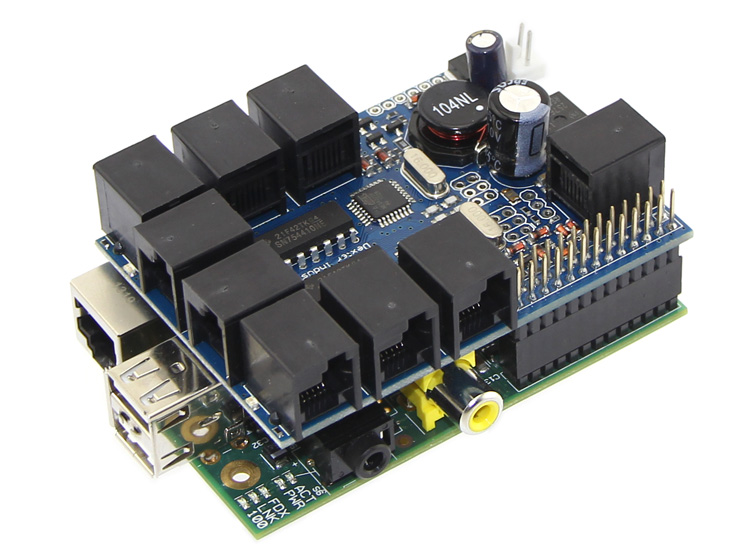
Web Interface Controller
Pythonfrom django.shortcuts import get_object_or_404, render
from django.template import RequestContext, loader
from django.http import HttpResponse, HttpResponseRedirect
from django.core.urlresolvers import reverse
from .models import Question
import sys
import subprocess as sp
photonOff="curl https://api.spark.io/v1/devices/310034000347343138333038/ledOff -d access_token= -d params=off"
photonRobot="curl https://api.spark.io/v1/devices/310034000347343138333038/ledGreen -d access_token= -d params=on"
photonSecurity="curl https://api.spark.io/v1/devices/310034000347343138333038/ledRed -d access_token= -d params=on"
photonComm="curl https://api.spark.io/v1/devices/310034000347343138333038/ledBlue -d access_token= -d params=on"
photonLife="curl https://api.spark.io/v1/devices/310034000347343138333038/ledGold -d access_token= -d params=on"
runRobot="ssh pi@brickpi 'python ~/MJ/LobotArm.py'"
runSMS="java -jar ~/lobot/jars/LobotSmsSender.jar"
runEmg="java -jar ~/lobot/jars/LobotEmgCall.jar"
def robot():
message="Activating Robotic Arm"
sp.call("~/scripts/speak.sh "+message,shell=True)
sp.call(photonRobot, shell=True)
sp.call(runRobot, shell=True)
def security(request):
message="Activating Security Cameras"
sp.call("~/scripts/speak.sh "+message,shell=True)
sp.call(photonSecurity, shell=True)
def comm():
message="Relaying communications"
sp.call("~/scripts/speak.sh "+message,shell=True)
sp.call(photonComm, shell=True)
sp.call(runSMS, shell=True)
def life():
message="Engaging Life Support Emergency procedures"
sp.call("~/scripts/speak.sh "+message,shell=True)
sp.call(photonLife, shell=True)
sp.call(runEmg, shell=True)
def off():
message="Shutting down"
sp.call("~/scripts/speak.sh "+message,shell=True)
sp.call(photonOff, shell=True)
def results(request, question_id, selected_choice_id):
question = get_object_or_404(Question, pk=question_id)
if selected_choice_id == "2":
return render(request, 'controls/security.html')
else:
return render(request, 'controls/results.html', {'question': question})
def vote(request, question_id):
question = get_object_or_404(Question, pk=question_id)
try:
selected_choice = question.choice_set.get(pk=request.POST['choice'])
except (KeyError, Choice.DoesNotExist):
# Redisplay the question voting form.
return render(request, 'controls/detail.html', {
'question': question,
'error_message': "You didn't select a choice.",
})
else:
if selected_choice.id == 1:
robot()
elif selected_choice.id == 2:
security(request)
elif selected_choice.id == 3:
comm()
elif selected_choice.id == 4:
life()
elif selected_choice.id == 5:
off()
else:
print "Invalid Result"
# Always return an HttpResponseRedirect after successfully dealing
# with POST data. This prevents data from being posted twice if a
# user hits the Back button.
return HttpResponseRedirect(reverse('controls:results', args=(question.id,selected_choice.id)))
def index(request):
latest_question_list = Question.objects.order_by('-pub_date')[:5]
template = loader.get_template('controls/index.html')
context = RequestContext(request, {
'latest_question_list': latest_question_list,
})
return HttpResponse(template.render(context))
def detail(request, question_id):
try:
question = Question.objects.get(pk=question_id)
except Question.DoesNotExist:
raise Http404("Question does not exist")
return render(request, 'controls/detail.html', {'question': question})
Emergency Calls
Javapackage Twilio.twilio;
import com.twilio.sdk.*;
import java.util.HashMap;
import java.util.Map;
public class LobotEmgCall {
/* Twilio REST API version */
public static final String APIVERSION = "2010-04-01";
public static void main(String[] args) {
/* Twilio AccountSid and AuthToken */
String AccountSid = "";
String AuthToken = "";
/* Outgoing Caller ID previously validated with Twilio */
String CallerID = "12407536576";
String ToCall = "";
String Url = "http://twimlets.com/message?Message%5B0%5D=Hello%20from%20my%20java%20application.&Message%5B1%5D=http%3A%2F%2Fcom.twilio.music.electronica.s3.amazonaws.com%2Fteru_-_110_Downtempo_Electronic_4.mp3";
/* Instantiate a new Twilio Rest Client */
TwilioRestClient client = new TwilioRestClient(AccountSid, AuthToken,
null);
// build map of post parameters
Map<String, String> params = new HashMap<String, String>();
params.put("From", CallerID);
params.put("To", ToCall);
params.put("Url", Url);
TwilioRestResponse response;
try {
response = client.request("/" + APIVERSION + "/Accounts/"
+ client.getAccountSid() + "/Calls", "POST", params);
if (response.isError())
System.out.println("Error making outgoing call: "
+ response.getHttpStatus() + "n"
+ response.getResponseText());
else {
System.out.println(response.getResponseText());
System.out.println("Lobot is making a call...");
}
} catch (TwilioRestException e) {
e.printStackTrace();
}
}
}
SMS Messenger
Javapackage Twilio.twilio;
import java.util.ArrayList;
import java.util.List;
import org.apache.http.NameValuePair;
import org.apache.http.message.BasicNameValuePair;
import com.twilio.sdk.TwilioRestClient;
import com.twilio.sdk.TwilioRestException;
import com.twilio.sdk.resource.factory.MessageFactory;
import com.twilio.sdk.resource.instance.Account;
public class LobotSmsSender {
/* Find your sid and token at twilio.com/user/account */
public static final String ACCOUNT_SID = "";
public static final String AUTH_TOKEN = "";
public static void main(String[] args) throws TwilioRestException {
TwilioRestClient client = new TwilioRestClient(ACCOUNT_SID, AUTH_TOKEN);
Account account = client.getAccount();
MessageFactory messageFactory = account.getMessageFactory();
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("To", ""));
params.add(new BasicNameValuePair("From", "+12407536576"));
params.add(new BasicNameValuePair("Body", "This is a message from Lobot. This is only a test!"));
messageFactory.create(params);
}
}
Photon Controller
Arduinoint greenPin = D7;
int redPin = D6;
int bluePin = D5;
int goldPin = D4;
void setup() {
pinMode(greenPin, OUTPUT);
pinMode(redPin, OUTPUT);
pinMode(bluePin, OUTPUT);
pinMode(goldPin, OUTPUT);
Spark.function("ledGreen", greenSwitch);
Spark.function("ledRed", redSwitch);
Spark.function("ledBlue", blueSwitch);
Spark.function("ledGold", goldSwitch);
Spark.function("ledOff", offSwitch);
}
int greenSwitch(String command)
{
if (command.equalsIgnoreCase("on"))
{
digitalWrite(greenPin, HIGH);
digitalWrite(redPin, LOW);
digitalWrite(bluePin, LOW);
digitalWrite(goldPin, LOW);
return 1;
}
return -1;
}
int redSwitch(String command)
{
if (command.equalsIgnoreCase("on"))
{
digitalWrite(greenPin, LOW);
digitalWrite(redPin, HIGH);
digitalWrite(bluePin, LOW);
digitalWrite(goldPin, LOW);
return 1;
}
return -1;
}
int blueSwitch(String command)
{
if (command.equalsIgnoreCase("on"))
{
digitalWrite(greenPin, LOW);
digitalWrite(redPin, LOW);
digitalWrite(bluePin, HIGH);
digitalWrite(goldPin, LOW);
return 1;
}
return -1;
}
int goldSwitch(String command)
{
if (command.equalsIgnoreCase("on"))
{
digitalWrite(greenPin, LOW);
digitalWrite(redPin, LOW);
digitalWrite(bluePin, LOW);
digitalWrite(goldPin, HIGH);
return 1;
}
return -1;
}
int offSwitch(String command)
{
if (command.equalsIgnoreCase("off"))
{
digitalWrite(greenPin, LOW);
digitalWrite(redPin, LOW);
digitalWrite(bluePin, LOW);
digitalWrite(goldPin, LOW);
return 1;
}
return -1;
}
import time
from BrickPi import *
BrickPiSetup() # setup the serial port for communication
BrickPi.MotorEnable[PORT_B] = 1 #Enable the Motor B
BrickPi.MotorEnable[PORT_C] = 1 #Enable the Motor C
BrickPi.MotorEnable[PORT_A] = 1 #Enable the Motor A
BrickPiSetupSensors() #Send the properties of sensors to BrickPi
BrickPi.MotorSpeed[PORT_B] = 0 #first setting all speeds to zero
BrickPi.MotorSpeed[PORT_C] = 0
BrickPi.MotorSpeed[PORT_A] = 0
BrickPiUpdateValues()
elapsed = time.time()
futureTime = time.time() + 10 #Run for 10 seconds
while elapsed < futureTime:
elapsed = time.time()
BrickPi.MotorSpeed[PORT_B] = -125 #Left
BrickPi.MotorSpeed[PORT_A] = -90 #open
BrickPiUpdateValues()
time.sleep(.1)
futureTime = time.time() + 15 #Run for 15 seconds
while elapsed < futureTime:
elapsed = time.time()
BrickPi.MotorSpeed[PORT_B] = 125 #Right
BrickPi.MotorSpeed[PORT_A] = 90 #close
BrickPiUpdateValues()
time.sleep(.1)
Comments