#include "mbed.h"
#include "CBuffer.h"
DigitalOut led1(LED1);
DigitalOut led4(LED4);
RawSerial pc(USBTX, USBRX); // tx, rx
RawSerial dev(D1, D0); // tx, rx for WIZwiki-W75000
#define ASYNC_DEBUG 0
#if ASYNC_DEBUG
////////////////////////////////////////////////////////////////////////////////////////////////
// mbed Async Debug
Timeout timer_buffer_debug;
CircBuffer<char> async_debugbufer(1024);
void print_debugbuffer()
{
char c=0;
while ( async_debugbufer.available() ) {
async_debugbufer.dequeue(&c);
pc.putc(c);
}
timer_buffer_debug.attach(&print_debugbuffer, 0.1);
}
#include <stdarg.h>
static char debug_line[64];
void mbed_async_debug(const char *format, ...)
{
va_list args;
va_start(args, format);
vsnprintf(debug_line, sizeof(debug_line), format, args);
int length = strlen(debug_line);
for (int i=0; i<length; i++)
async_debugbufer.queue(debug_line[i]);
va_end(args);
}
// mbed Async Debug Print, You can move below line to mbed-src.
void (*dbg_f)(const char *format, ...);
extern void (*dbg_f)(const char *format, ...);
#endif
void dev_recv()
{
led1 = !led1;
while(dev.readable()) {
pc.putc(dev.getc());
}
}
void pc_recv()
{
led4 = !led4;
while(pc.readable()) {
dev.putc(pc.getc());
}
}
int main()
{
for (int i=0; i<10; i++)
{
led1 = !led1;
led4 = !led4;
wait(0.1);
}
pc.baud(115200);
dev.baud(9600);
pc.printf("Serial Passthrough Started. \r\n");
#if ASYNC_DEBUG
dbg_f = &mbed_async_debug;
timer_buffer_debug.attach(&print_debugbuffer, 0.1);
#endif
pc.attach(&pc_recv, Serial::RxIrq);
dev.attach(&dev_recv, Serial::RxIrq);
while(1) {
wait(1);
}
}
Serial passthrough using WIZwiki-W7500 and gainspan-WiFi-mod
I can issue at-command-sets to the WiFi module with WIZwiki-W7500's serial.
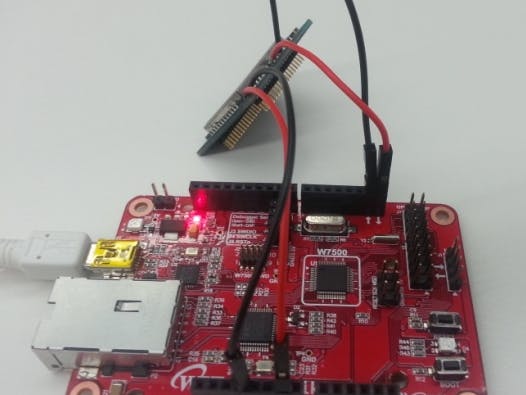
Comments